Instructions
- Problem Set Self Assessment
- Weekly Participation
- Reflection
- Learning Plan
- Quiz/Exam Revisions
- Programming Assignments
Problem Set Self Assessment
- On Canvas, go to the Problem Set Solutions folder under the Files tab to view the solution for the relevant problem set.
- From the Assignments tab on Canvas, click on the problem set to self assess.
- Click on the link "Submission Details," which can be found either on the right side bar of the page, or at the bottom of the page.
- Click the link "View Feedback" and enter full screen mode of the Canvas editor.
- Use the point annotation to make comments for each problem, based on my solutions:
- If correct, describe any relevant skills you have demonstrated.
- If incorrect, describe the type of error, and how you might improve in the future.
- Exapmles of errors:
- Incorrectly implemented [some linear algebraic operation]
- Algebra error
- Did not correctly interpret the problem description into math
- Misunderstood/Misapplied [quantum concept]
- Did not read the problem carefully
- Examples of improvements:
- Start earlier to have time to review after time away to see with fresh eyes
- Read each problem carefully during rough draft phase and again in pset phase
- Spend more time practicing with logarithms
- Otional: Add the most important self-feedback to you Feedback List in your personal OneNote section.
- If there are problems you would like additional feed-back on, or if you have a problem-set specific question, after exiting the Canvas editor, in the "Add a comment" box on the right side-bar, write which problem parts you would like additional feedback on, or ask a question, or both. I or a TA will respond to your questions.
- You're done!
Weekly Participation
- If correct, describe any relevant skills you have demonstrated.
- If incorrect, describe the type of error, and how you might improve in the future.
- Exapmles of errors:
- Incorrectly implemented [some linear algebraic operation]
- Algebra error
- Did not correctly interpret the problem description into math
- Misunderstood/Misapplied [quantum concept]
- Did not read the problem carefully
- Examples of improvements:
- Start earlier to have time to review after time away to see with fresh eyes
- Read each problem carefully during rough draft phase and again in pset phase
- Spend more time practicing with logarithms
- Otional: Add the most important self-feedback to you Feedback List in your personal OneNote section.
You are expected to participate weekly in the class in several modes. You will fill out a weekly check-in form to let me know how you have participated each week. Weekly participation will earn you participation points.
Exit Tickets
After each class, you have 24 hours to write an exit ticket on OneNote. Your exit ticket should let me know if there is anything that you were confused about, anything you would like to see more of, or a general question about the material. You can also put a star next to someone else's question that you second. (Questions with the most stars are the ones I will prioritize.)
Choose Your Own Adventure Participation
To get full weekly participation credit, you must also participate in one or more of the additional ways each week:
- Come to office hours.
- Ask questions during class
- Meet with classmates outside of class to work on problems/study.
- Ask a question or answer a question on Teams
- Attend tutoring hours
- Alert me to typos in any of my materials. Everyone's learning will be hampered if my material is confusing because of a typo!
- Have another idea for participation? Please come discuss with me.
While you only need to do one to get credit, I hope you will do several, as they will contribute to your learning!
Reflections
Your reflections should be completed in your personal Reflection section of the course OneNote. (This section is visible to me, but not your classmates.) There is a page for the reflection that you should fill out. For the first reflection, see the prompt. Later prompts are as follows:
To complete your reflection, you should read over your prior reflection, and consider your progress since your last reflection, as demonstrated by your problem sets, quizzes, quiz revisions, and in-class group participation. Please write a paragraph or two about each topic, following the prompts. If you would like to vary the format of your reflection to make it more useful for you, that is fine, as long as you are reflecting critically on your learning process and progress and your interactions with others in your group.
- Process
- Reflect on your learning process since your last reflection. What has supported your learning, and what has hampered your learning? Describe your progress towards process sub-goals you laid out in your prior reflection. Were you able to make progress in incorporating the feedback items from your Feedback List that you are currently focused on? Please mark in bold any feedback items that you would like to focus on for the next few weeks, and unbold any that you are no longer focused on. If needed, describe some process sub-goals to focus on for the next few weeks.
- Objectives
- Reflect on your progress towards the learning goals of the course. What are the most important skills and/or understanding that you demonstrated? Take a look at your quizzes/problem sets/exams to see, and make a list of the most important. What are you still struggling with? How will your progress towards your goals inform or change your process moving forward?
- Group Work
- Look back at your prior group work reflection - have you made progress in the areas that you wanted to focus on? What did you do in group work in the past weeks to actively engage each member of your group? What could you have done to better engage with each member and to make sure each member had the space to contribute and learn? What do you plan to do in the future to make group work a more productive learning experience for each person in your group? Was there anything you found particularly valuable or frustrating about group work recently? If someone in your group is creating an environment in which it is challenging to learn, please let me know.
Mid-semester and Final Reflection
These reflections take the same format as a standard reflection, but instead of focusing on the past couple weeks, you should reflect on your progress throughout the semester, and you should think about your goals moving forward for the second half of the semester (mid-semester) or your life more generally (final).Quiz/Exam Revisions
- Click on the "Grades" tab in Canvas, and then click on the quiz/exam you want to revise.
- Look at your previous solutions and the feedback comments to try to understand what you did not fully demonstrate understanding in your first attempt. Looking over class notes and the problem set solutions for similar problems might be helpful. You can also discuss with Prof. Kimmel, or with a peer who has already taken the quiz. (If you discuss with a peer, please do not look at their submission, instead, you should discuss the concepts with them generally, or can go over similar problems in more detail.)
- If the feedback is something you want to keep in mind moving forward, copy it to your Feedback List on OneNote.
- Retake the quiz/exam to demonstrate your understanding/skill. If you previously submitted a pdf, you can edit/make comments over the original pdf and re-upload. This will also make it easier for us to see your changes.
- Click on the "Grades" tab in Canvas again, and in the "Add a Comment" field to the right or below the Quiz Results, explain:
- What did you not yet fully understand when you last took the quiz?
- How has your understanding improved and how did you demonstrate that knowledge in your revision?
- Moving forward, how will you ensure that you continue to demonstrate understanding of this concept(s)/skill(s)?
Programming Assignments
General Instructions
As with the problem sets, to get full credit for the Rough Draft part of the programming assignment, you should submit something that shows you have spent at least an hour or two working on the assignment.
For the main assignment, you should submit a .zip file containing the code, and any other files needed to run the code.
In addition, the .zip file should contain a file (in format .txt, .doc, or .pdf, etc.) called "Assessment" that answers the following questions:
- Harm/Benefit Analysis: [Describe who is most likely to be harmed and who is most likely to benefit from this code, if it is used in the real world. Is the good greater than the harm? Would you be willing to implement it?]
- Correctness Assessment: [Does the code compile? Does the code run without errors? Does the code output the correct answer on test cases? What edge cases have you tried? What issues are you aware of?]
- Modularity/Extensibility/Flexibility: [Describe how you broke up your code into methods or classes in order to make the code easier to organize/reuse. Is there anything that you hard-coded that would present challenges if the input were modified, or if additional functionality was required?]
- Readability: [Is your code commented? Did you choose variable and method names that help the reader understand their purpose?]
- Effort: [Describe the effort (time, approaches you took when stuck, resources you used including people you worked with) that you put into the assignment. If you could go back and do it again, how would your approach change?]
For sufficient effort, which we will judge by your response on Effort, and by looking at the code that you did turn in, you will earn 3 pts. For correct, extensible, readable code, that meets assignment specifications, you will earn another 3 points.
Programming Assignment 1
Read the General Instructions and Honor Code.
The number of school-aged children in Vermont has been falling, and it is getting expensive to keep open schools that have only a few students. To deal with this, many towns are consolidating schools (combining students in two neighboring towns into one school). Data on K-12 school locations is publicly available via the Vermont Open Geodata Portal. In this assignment, you will write a program that finds the closest pair of K-12 schools in Vermont. This program could be used to suggest schools as candidates for mergers.
Complete the method ClosestPoints
from this
zip file containing the data and starter classes. Your code should run in O(nlogn) time. You should feel free to add additional methods or classes as appropriate for clarity or elegance.
To run from the command line, open a terminal in the directory where ClosestSchools is located. Type
javac ClosestSchools/*.java
to compile, and java -cp . ClosestSchools.Main
to run. (-cp allows you to tell java where to look for the compiled code, and . tells it to look in the current directory)
Programming Assignment 2
Read the General Instructions and Honor Code.
Create a class Huffman in Java with a method encode
that takes in a String. The String can contain any characters (including lower case letters, upper case letters, and the following punctuation: commas, periods, exclamation points, question marks, and apostraphes). It should output a new String containing only 0's and 1's that is the encoding of the original String using Huffman's algorithm. You should weight each letter or symbol according to how often it appears in your input String. encode
should also print a dictionary that can be used to translate between binary and English.
t - 001
d - 10
o - 0001
⋮
There are lots of versions of this code online, so please do not search for Huffman's Algorithm on the internet. However, you can look up how to do subtasks like creating trees or doing a tree traversal.
You can either use a min-heap/priority queue (you can use, for example, Java's PriorityQueue class), or you can just do a slower search for the smallest weight trees.
Here is some starter code: starter code.
In the comments at the top of Main.java, please include your name, the approximate number of hours you worked on the assignment, and the names of anyone you worked with.
To run from the command line, open a terminal/shell in the directory where Huffman.java is located. Type
javac Huffman.java
to compile, and java -cp . Huffman
to run. (-cp allows you to tell java where to look for the compiled code, and . tells it to look in the current directory)
To use Eclipse, see instructions below.
FAQ
- Do I need to encode lower case letters? Upper case letters? Spaces? Yes. Yes. Yes.
- Do I have to write a binary tree object from scratch? No! You can use the one you created in 201, or you can look up an example on the internet to base your code on. In either case, you should mention the place you got your code from.
- Do I have to write a tree traversal from scratch? No! You can use the one that you created in 201, or you can look up an example on the internet to base your code on. In either case, you should mention the place you got your code from.
- Do I have to implement a custom Comparator if I use the PriorityQueue class? Yes. Here is an example that seems like it would be helpful.
- Wait...do I have to use a tree? No - there are ways that you could implement this algorithm without implementing a tree.
Programming Assignment 3
Read the General Instructions and Honor Code.
[This scenario is based on a post-pandemic dream when you are all back at school!] You are at 75 Shannon St. and you are hungry! You would like to walk to a restaurant in town and get there using the shortest possible route. You will use the Bellman Ford algorithm to find the shortest route. (See what I did there? After doing this, you will have implemented a divide and conquer, a greedy, and a dynamic programming algorithm!) You will again use data from the Vermont Open Geodata Portal.
Complete the methods ShortestDistance
and ShortestPath
from the Class Graph.java
in this zip file zip file containing the data and starter classes. You should feel free to add additional methods or classes as appropriate for clarity or elegance.
To run from the command line, open a terminal in the directory where ClosestSchools is located. Type
javac ShortestMiddPath/*.java
to compile, and java -cp . ShortestMiddPath.Main
to run. (-cp allows you to tell java where to look for the compiled code, and . tells it to look in the current directory)
FAQ
- What should the output of the path look like? I'm not too picky, but something like, a list of street names, separated by commas, that you could follow to get from 75 Shannon to your destination.
- How do I find other places to test? Go to Vermont Geodata Portal, and click on the road that your destination on. You next need to figure out if your destination is closest to the start or end id. The problem is that you don't know which end of the road is the start and which is the end, so go to a neighboring road and see which start id or end id it has in common.
- Is the adjacency list a reverse or regular list? As discussed in the code, the graph is undirected, so the adjacency list is both a regular and reverse list at the same time.
- I'm getting repeated street names - what is going on? As long as you understand why they are repeated, you can leave them in the output.
Using Eclipse
You do not have to use Eclipse, but if you would prefer to do so, here are some instructions (modified for the first assignment, you should hopefully be able to adjust for the others). If it is not working, please reach out to Robert Lichenstein, our fearless ASI.
- Create a new Eclipse Project
- File -> New -> Java Project
- Enter "Closest Schools" as Project Name. Hit finish.
- Click "Don't Create" for if it asks to generate module-info.java
- Insert Class Files
- Right-Click on the Project Folder in the left window
- New-> Class -> Name the file "test" (delete it later)
- You should now see a default package (or similar) folder inside the /src folder.
- Copy Main.java and School.java from ClosestSchools.zip, paste them in the default package folder inside src.
- If it gives you an error on the line "package Closest Schools;" just delete the line.
- Insert Data Files
- Copy the Data folder from the ClosestSchools.Zip
- select the /src folder in the left window, under your project
- paste the Data folder here.
- Modify the file path on line 36 of Main.java to read: "Data/VT_School_Locations__K12(1).csv"
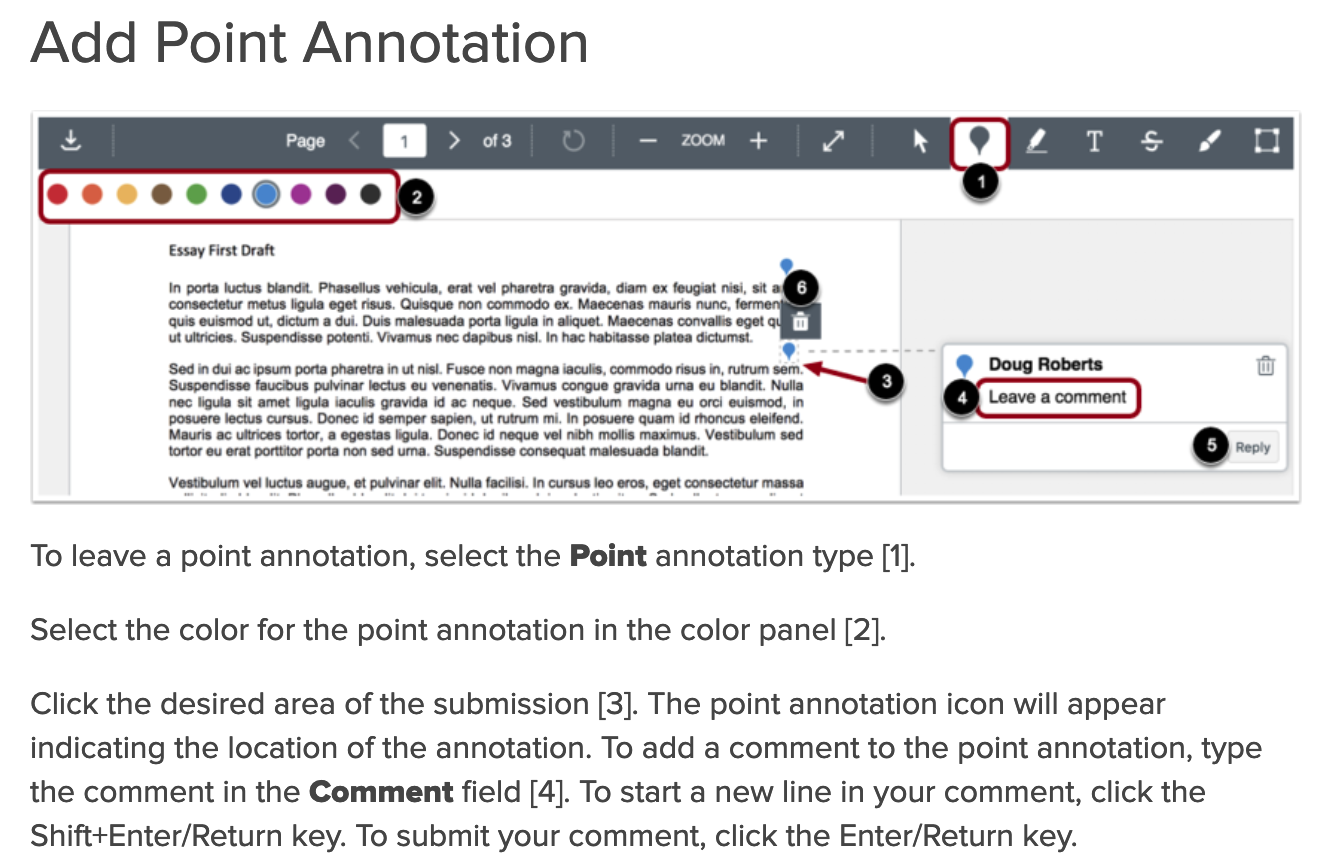